This post is here to help you understand implicit vs explicit data conversions in SQL Server.
What is Data Conversion?
Data conversion involves transforming data from one type to another. SQL Server facilitates this through two primary methods:
Implicit Conversion
> Occurs automatically when SQL Server can safely convert one data type to another without the risk of data loss or errors.
> Example: Converting an INT
to a FLOAT
happens seamlessly.
Explicit Conversion
> Requires manual intervention using functions like CAST
or CONVERT
.
> Example: Converting a VARCHAR
to a DATETIME
demands explicit instructions, especially when formats are non-standard.
SQL Server Data Type Conversion Chart
SQL Server provides a detailed conversion chart that outlines compatible data types and the direction of conversion:
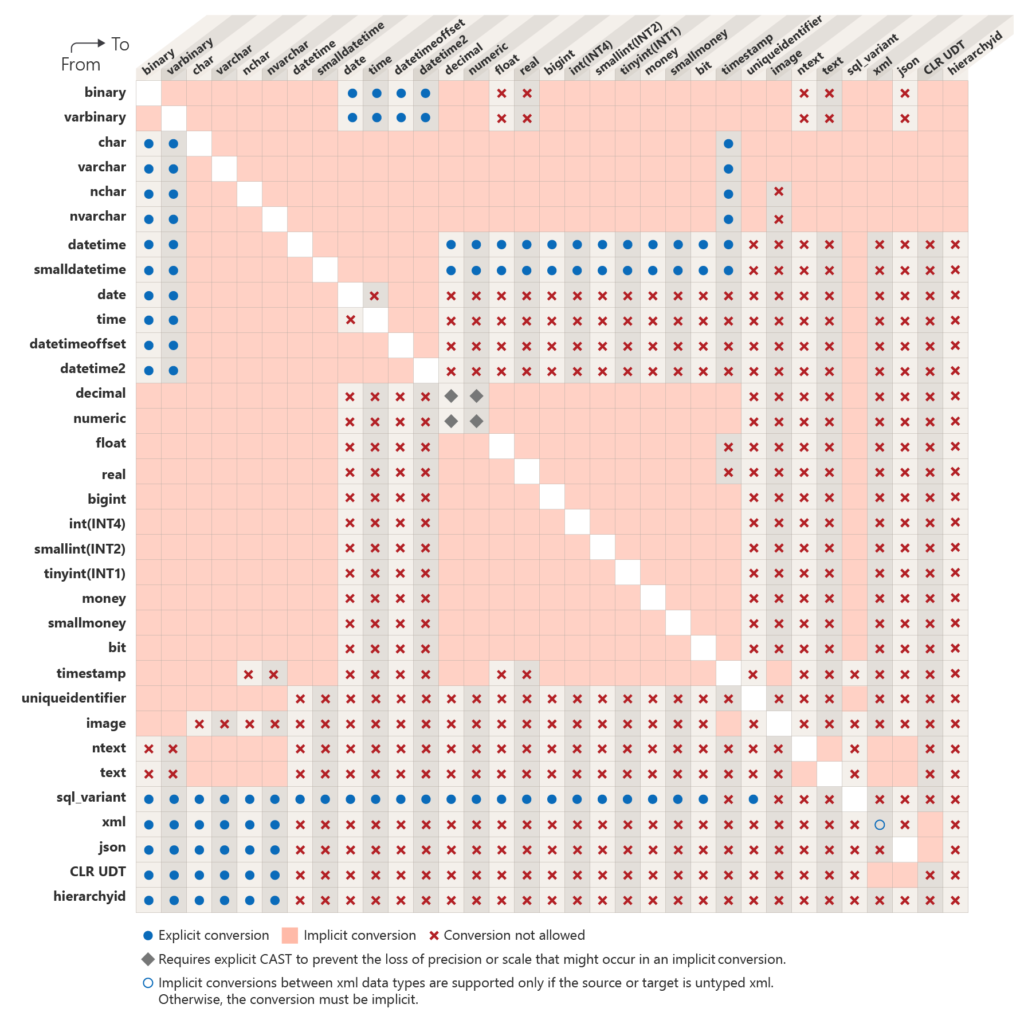
For the most accurate and updated version, refer to the official SQL Server Data Type Conversion Documentation.
Interpreting the Conversion Chart
> Converting an INT
to a FLOAT
is implicit and happens automatically without errors.
> Converting a VARCHAR
to a DATETIME
requires explicit conversion, as mismatched formats may cause errors.
Examples of Explicit Conversions
Explicit conversions offer greater control over data type transformations, ensuring precision and avoiding errors. Here are practical examples:
Valid Explicit Conversion:
Converting a VARCHAR
containing a valid date to a DATETIME
:
DECLARE @dateString VARCHAR(10) = '2025-01-25'; SELECT CAST(@dateString AS DATETIME) AS ConvertedDate; SELECT CONVERT(DATETIME, @dateString, 120) AS ConvertedDateWithStyle;
In the above example:
> CAST
converts the VARCHAR
to DATETIME
directly.
> CONVERT
offers additional formatting options using style codes (e.g., 120
for yyyy-mm-dd
).
Explicit Conversion with Errors
When the VARCHAR
does not represent a recognizable date format, conversion fails:
DECLARE @invalidDate VARCHAR(10) = 'invalid'; SELECT CAST(@invalidDate AS DATETIME); -- Results in an error /* Msg 241, Level 16, State 1, Line 3 Conversion failed when converting date and/or time from character string. */
Best Practices for Data Conversion
Validate Input:
Ensure input data is clean and adheres to expected formats before conversion.
Use Explicit Conversion for Clarity:
Even when implicit conversion is possible, explicit conversion improves code readability and reduces ambiguity.
Handle Errors Gracefully:
Use TRY_CONVERT
or TRY_CAST
to avoid runtime errors in case of invalid data:
DECLARE @invalidDate VARCHAR(10) = 'invalid'; SELECT TRY_CAST(@invalidDate AS DATETIME) AS SafeConversion;
This approach returns NULL
instead of throwing an error.
Hope this random post was useful!
Leave a Reply