If you need to create folders or subfolders in PowerShell only when they don’t already exist, this quick script will help. The script uses Test-Path
to check if the folder exists before running the New-Item
command.
This snippet is ideal for anyone searching for a fast and reliable way to ensure directories are created without errors.
PowerShell to Create a Folder If Not Exists
Here’s the code to create a folder (e.g., C:\temp\demo
) which tests if it exists first before creating:
# create folder if not exists .ps1 $path = "c:\temp\demo" If(!(Test-Path $path) ){ New-Item -ItemType Directory -Force -Path $path }
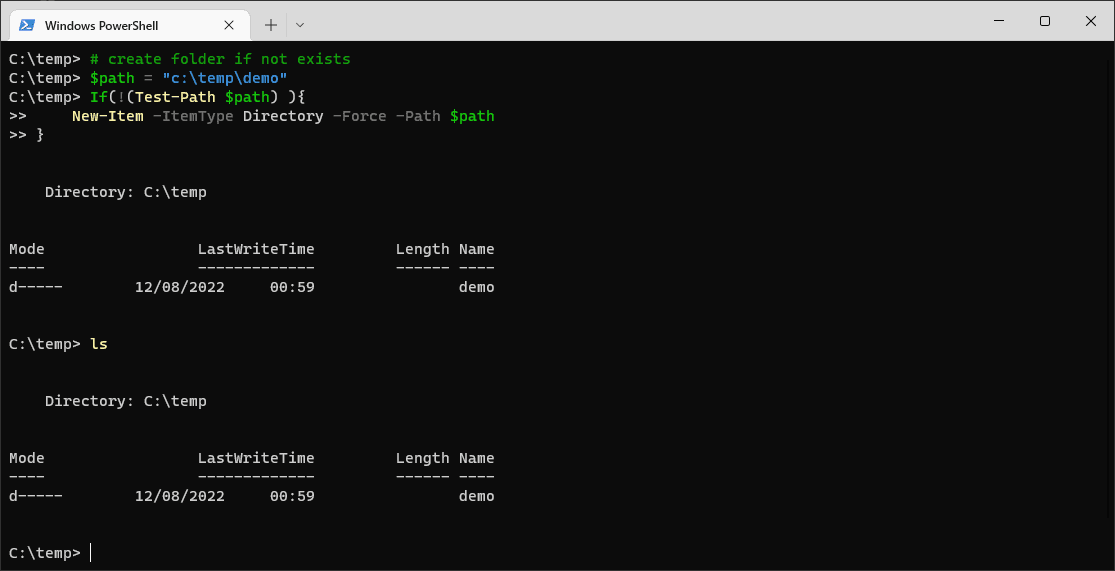
How It Works
> $path
: Set this to the directory you want to create.
> Test-Path
: Checks if the folder exists.
> New-Item
: Creates the folder if Test-Path
returns false.
> -Force
: Ensures the command runs even if parts of the directory (like C:\temp
) don’t exist yet.
More Tips
> Nested Directories: PowerShell will create parent directories automatically with the -Force
parameter. For example, if C:\temp
doesn’t exist, it will be created alongside C:\temp\demo
.
> Custom Paths: Update the $path
variable to match the folder you need for your use case.
> Reusable Code: Save this script as a .ps1
file for quick reuse.
> Error Handling: Wrap the script in a try-catch
block if you want to handle any potential errors gracefully.
Check out my PowerShell Blog Category for more random tips like this one!
Leave a Reply