In this post, we will explore how to use PowerShell to view event logs. We will use the Get-EventLog
command to accomplish this, listing event types available and then show recent events.
1. List Available Event Log Types
To display all event log types on a system, run Get-EventLog -List
as shown below:
# Get event types PowerShell Get-EventLog -List
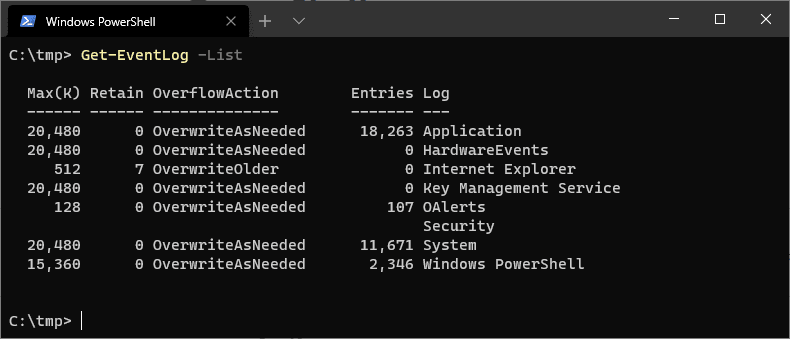
This command returns event categories such as System, Security, and Application. You can then specify a particular log type using the -LogName
parameter in subsequent commands.
2. Get Most Recent Events
To retrieve the 100 most recent events from the System log, run the following:
# Get most recent Windows events PowerShell Get-EventLog -LogName System -Newest 100
For a high-level view of frequent errors, group and count the newest 1000 error events from the Application log:
# Get most recent application events by count Get-EventLog -LogName Application -Newest 1000 -EntryType Error | Group-Object -Property Source -NoElement | Sort-Object -Property Count -Descending
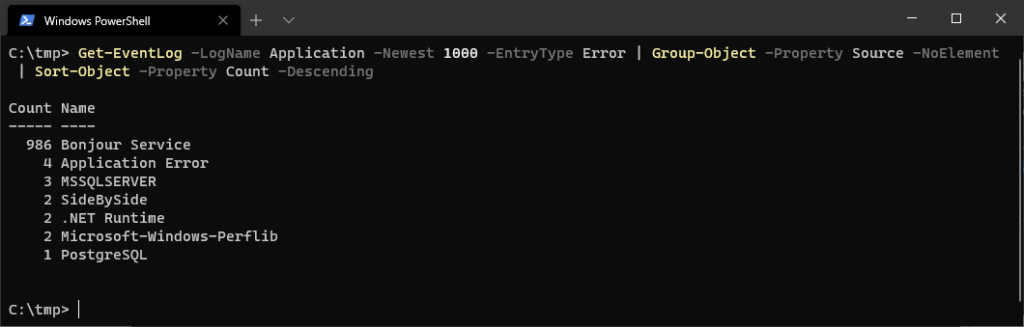
This reveals recurring error sources, helping identify persistent issues.
3. Get Events Between Specific Dates
To retrieve critical and error events within a date range, use the Get-WinEvent
cmdlet in a PowerShell script:
param( [DateTime]$StartDate, [DateTime]$EndDate ) # Get all critical and error events from the Windows event logs Get-WinEvent -FilterHashtable @{ LogName = 'System, Application'; Level = 1, 2; StartTime = $StartDate; EndTime = $EndDate }
Replace START_DATE
and END_DATE
with your desired date range:
.\Get-CriticalAndErrorEvents.ps1 -StartDate '2021-01-01' -EndDate '2021-12-31'
This script filters events by:
– Date Range: Defined by your start and end dates
– Log Name: System and Application logs
– Level: Critical (1) and Error (2) events
Using PowerShell to explore and filter event logs offers a powerful way to troubleshoot and monitor system health. These commands and scripts will help you pinpoint issues and understand system behavior more effectively. Hope all this helps!
Leave a Reply