This post contains an advanced guide for creating and managing files and folders in PowerShell. It builds on the basics introduced in my previous post, PowerShell Guide: Creating Folders and Files, and includes more techniques useful for a DBA or Sysadmin.
Contents:
1. Creating New Files and Folders
2. Batch Creation of Files and Folders
3. Creating Files and Folders Conditionally
4. Automating Nested Folder Structures
5. Checking for Files Older Than 30 Days
1. Creating New Files and Folders
New-Item
is the PowerShell cmdlet to create the new folder or file, we just need to amend the ItemType
parameter choosing Directory or File whichever is needed:
# create new folder New-Item -ItemType Directory -Name Test_Stuff # create new file New-Item -ItemType File -Name Test_File.txt
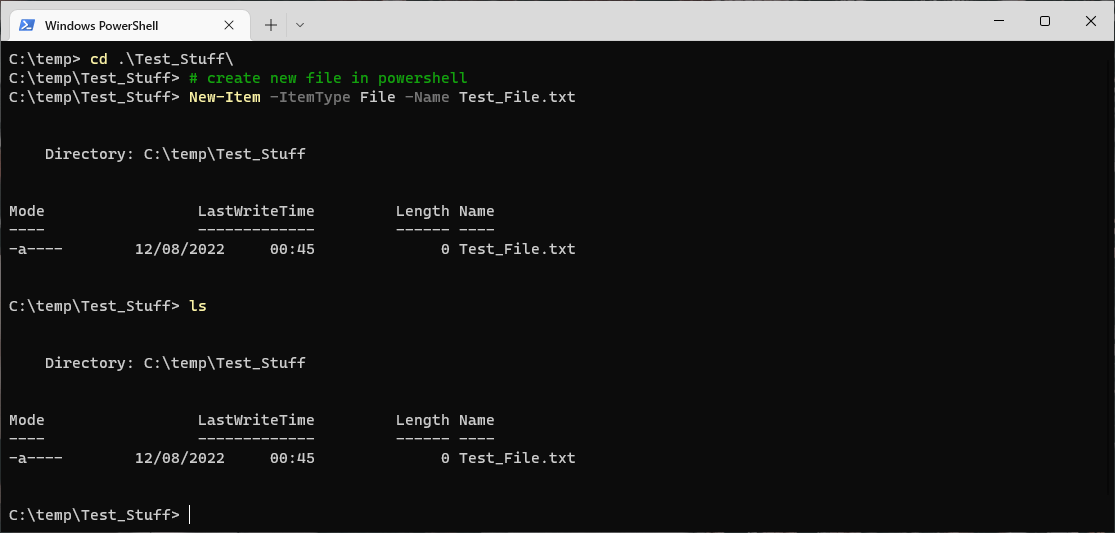
2. Batch Creation of Files and Folders
When you need to create multiple files or folders in one go, PowerShell’s pipeline and looping capabilities come in handy:
# Create multiple folders "Folder1", "Folder2", "Folder3" | ForEach-Object { New-Item -ItemType Directory -Name $_ -Path C:\Projects } # Create multiple files "File1.txt", "File2.txt", "File3.txt" | ForEach-Object { New-Item -ItemType File -Name $_ -Path C:\Logs }
This approach ensures you can efficiently create multiple resources with minimal effort.
3. Conditional File Creation
Sometimes, you need to check if a file or folder exists before creating it. Use the Test-Path
cmdlet to conditionally create resources:
# Create a folder if it doesn't exist $path = "C:\Projects\Logs" If (!(Test-Path -Path $path)) { New-Item -ItemType Directory -Path $path } # Create a file if it doesn't exist $filePath = "C:\Logs\LogFile.txt" If (!(Test-Path -Path $filePath)) { New-Item -ItemType File -Path $filePath }
4. Automating Nested Folder Structures
PowerShell can easily handle the creation of nested folders with the -Force
parameter, which ensures all intermediate directories are created:
# Create nested folder structure New-Item -ItemType Directory -Path C:\Projects\2025\January\Reports -Force
You can combine this with scripting to dynamically generate paths and automate folder creation for various use cases.
5. Checking for Files Older Than 30 Days
For maintenance tasks like cleaning up old files, you can use PowerShell to identify and act on files based on their age:
# Get files older than 30 days $path = "C:\Logs" Get-ChildItem -Path $path -File | Where-Object { $_.LastWriteTime -lt (Get-Date).AddDays(-30) } | ForEach-Object { Write-Output "Deleting: $($_.FullName)" Remove-Item $_.FullName }
This script locates and deletes all files in the specified directory that haven’t been modified in the last 30 days.
Leave a Reply