The PowerShell script included in this blog post checks if a specific folder exists and then creates it if it does not already exist.
It does this by using the Test-Path command to check for the existence of the folder and the New-Item command to create it if it doesn’t exist. The script can be modified to check for and create any desired folder by changing the specified path.
The following is included in this PowerShell demo:
– Using Test-Path to Check if a Folder Exists
– Creating a Folder with New-Item
– Modifying the Script for Others
Using Test-Path to Check if a Folder Exists
The first step in this script is to use the Test-Path
command to check if the desired folder already exists. The Test-Path
command returns a boolean value indicating whether the specified path exists.
To check if a folder exists using PowerShell, we can use the following syntax:
# Check if folder exists $path = "c:\temp\" If(!(test-path $path))
Here, we are storing the path of the folder we want to check in the $path
variable. The !
operator negates the boolean value returned by Test-Path
, so the If
statement will only execute if the folder does not exist.
Creating a Folder with New-Item
To create a new folder, we can use the New-Item
command with the -ItemType
parameter set to Directory
. The -Force
parameter ensures that the command will overwrite any existing files with the same name and the -Path
parameter specifies the location where the new folder will be created.
# create a new folder New-Item -ItemType Directory -Force -Path $path
Modifying the Script for Others
To use this script to check for and create a different folder, simply modify the $path
variable to the desired location. For example, to check for and create the c:\myfolder\
folder, you would use the following script:
# create folder if not exists $path = "c:\myfolder\" If(!(test-path $path)) { New-Item -ItemType Directory -Force -Path $path }
By modifying the $path
variable, you can use this script to check for and create any folder you need.
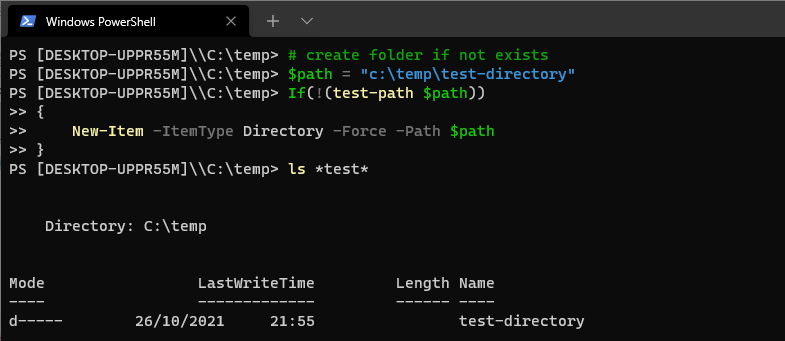