How to Delete Files in PowerShell
How to Delete Files in PowerShell
This is a post on how to delete files in PowerShell, which will be useful when you need to delete files as part of a script in Windows.
This post covers the following:
# PowerShell: Delete a File
# PowerShell: Delete a Folder
# PowerShell: Delete Files in Subfolders Recursively
I have a similar post on How to Create Files & Folders in PowerShell if of interest, and more random tips can be found in the PowerShell Tips Tag.
PowerShell: Delete a File
Remove-Item is the cmdlet to remove a directory or file in PowerShell. We can delete files within the current directory, or add the path parameter.
I’m showing some syntax variations below. If you are passing a directory with spaces in the name then you will have to add quotes to the path.
# Delete file in current directory Remove-Item -Name testFile.txt # Delete file in specified directory rm -Path C:\temp\demoFolder\testFile.txt # Delete all files in specified directory with .tmp file extension rm "C:\temp\*.tmp"
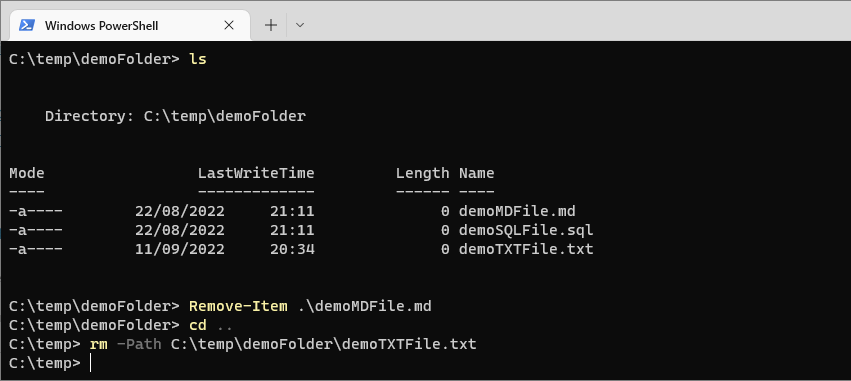
PowerShell: Delete a Folder
We do not need to change any parameters to delete a file, just run the same command as above. The consideration when deleting folders is the files within them.
The following demo script first deletes a folder within the current directory, and the second part checks if folders exist before deleting them.
# Delete a folder in current directory rm .\demoFolder\ # Delete folder if exists, force delete all files in folder # Does not delete files in sub-directories $path = "c:\temp\demoFolder" If((Test-Path $path) ){ rm $path -Force -Recurse }
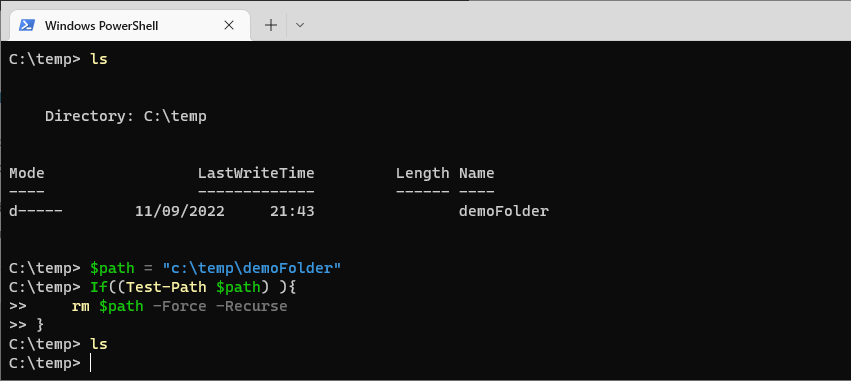
PowerShell: Delete Files in Subfolders Recursively
For when you need to delete files in a folder and subfolders, the script below should help. Amend path and file type wildcard as needed.
# Delete all .txt files in folder & subfolders $path = "c:\temp\demoFolder" If((Test-Path $path) ){ Get-ChildItem $path -Include *.txt -Recurse | rm }
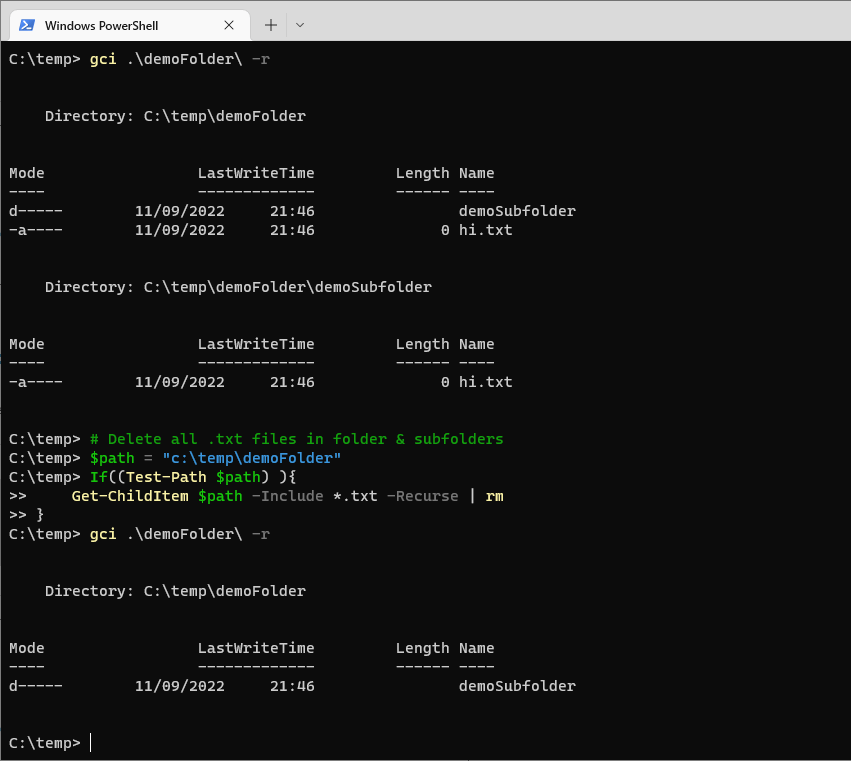
Last additional note on this, I haven’t used gci
within any of the PowerShell scripts above in this post. It’s an Alias of Get-ChildItem.
0 Comments